Last Updated on March 3, 2022 by Ria Pathak
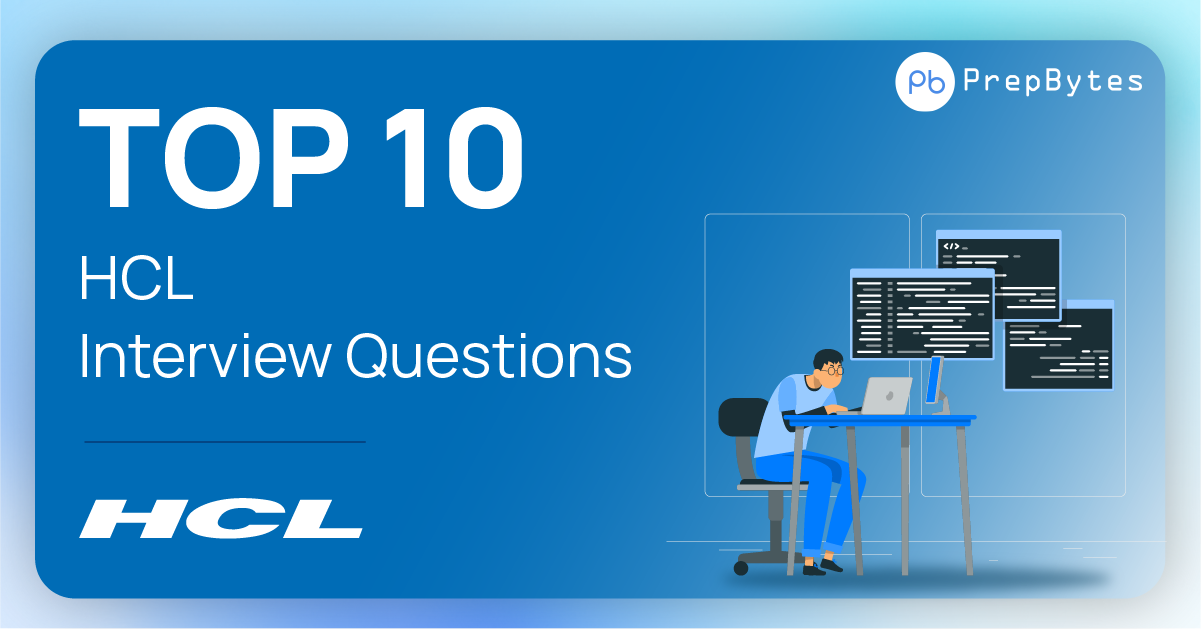
Want to work for HCL? Here we discuss some of the commonly asked interview questions.
What is the purpose of “register” keyword in C?
Register keyword declares register variables which have the same functionality as that of the auto variables.
The only difference is that the compiler tries to store these variables in the register of the microprocessor if a free register is available. This makes the use of register variables to be much faster than that of the variables stored in the memory during the runtime of the program. If a free register is not available, these are then stored in the memory only.
Usually few variables which are to be accessed very frequently in a program are declared with the register keyword which improves the running time of the program. An important and interesting point to be noted here is that we cannot obtain the address of a register variable using pointers.
To specify the storage class for a variable, the following syntax is to be followed:
register char b = âGâ;
What is Mesh topology?
Mesh topology: It is a point-to-point connection to other nodes or devices. All the network nodes are connected to each other. Mesh has n(n-1)/2 physical channels to link n devices.
There are two techniques to transmit data over the Mesh topology, they are:
- Routing: In routing, the nodes have a routing logic, as per the network requirements. Like routing logic to direct the data to reach the destination using the shortest distance. Or, routing logic which has information about the broken links, and it avoids those nodes etc. We can even have routing logic, to re-configure the failed nodes.
- Flooding: In flooding, the same data is transmitted to all the network nodes, hence no routing logic is required. The network is robust, and the its very unlikely to lose the data. But it leads to unwanted load over the network.
What is critical section?
Each process has a segment of code called critical section in which a process may be changing common variables, updating a shared table, writing a shared file and so on. The important feature of the system is that , when one process is executing in their critical sections , no other processes to be allowed to execute in its critical sections at the same time.
Each process must request permission to enter its critical section. The section of code implementing this request is the Entry section.
The critical section is followed by exit section.
The remaining code is remainder section.
Discuss TCP/IP modelTCP/IP model has following for layers:
Layer 1: Host-to-network Layer
- Lowest layer of the all.
- Protocol is used to connect to the host, so that the packets can be sent over it.
- Varies from host to host and network to network.
Layer 2: Internet Layer
- Selection of a packet switching network which is based on a connectionless internetwork layer is called a internet layer.
- It is the layer which holds the whole architecture together.
- It helps the packet to travel independently to the destination.
- Order in which packets are received is different from the way they are sent.
- IP (Internet Protocol) is used in this layer.
- Delivering IP packets
- Performing routing
- Avoiding congestion
Layer 3: Transport Layer
- It decides if data transmission should be on parallel path or single path.
- Functions such as multiplexing, segmenting or splitting on the data is done by transport layer.
- The applications can read and write to the transport layer.
- Transport layer adds header information to the data.
- Transport layer breaks the message (data) into small units so that they are handled more efficiently by the network layer.
- Transport layer also arrange the packets to be sent, in sequence.
Layer 4: Application Layer
The TCP/IP specifications described a lot of applications that were at the top of the protocol stack.
Two end to end protocols are:
- TCP(Transmission Control Protocol): It is a reliable connection-oriented protocol which handles byte-stream from source to destination without error and flow control.
- UDP(User-Datagram Protocol): It is an unreliable connection-less protocol that do not want TCPs, sequencing and flow control. Eg: One-shot request-reply kind of service.
What are constructors and destructors in C++?
Constructors: A class constructor is a special member function of a class that is executed whenever we create new objects of that class. A constructor will have exact same name as the class and it does not have any return type at all, not even void. Constructors can be very useful for setting initial values for certain member variables.
Constructor Example:
class Date {
Date(int, int, int) { //constructor
}
Date today = Date(23, 6, 1983); //constructor is called here
Date xmas(25, 12, 1990); //abbreviated form
Date myBirthday; //error : initializer missing
Date release(10, 12); // error : 3rd argument missing and there is no constructor with 2 arguments
Destructor: Destructor is a member function which destructs or deletes an object when an object goes out of scope. Destructors have same name as the class preceded by a tilde (~).Destructors donât take any argument and donât return anything Destructor can be very useful for releasing resources before coming out of the program like closing files, releasing memories etc.
Example of destructor:
class String {
private:
char * s;
int size;
public:
String(char * ); // constructor
~String(); // destructor
};
String::String(char * c) {
size = strlen(c);
s = new char[size + 1];
strcpy(s, c);
}
String::~String() {
delete[] s;
}
What are thread pools?
The general idea behind thread pools to create a number of threads at process startup and place them into a pool , where they sit and wait for work. Whenever a need occurs for a new thread for a task , a thread is awakened from this pool . Once the task assign to thread is completed it returns to pool and awaits more work. In case pool contains no available thread , the task waits until one becomes free.
Thread pool has following benefits:
- Servicing a request with an existing thread is usually faster than waiting to create a thread.
- A thread pool limits the number of threads that exist at any one point. This is particularly important on systems that cannot support a large number of concurrent threads.
Search an element in a sorted and rotated array
An element in a sorted array can be found in O(log n) time via binary search. But suppose we rotate an ascending order sorted array at some pivot unknown to you beforehand. So for instance, 1 2 3 4 5 might become 3 4 5 1 2.
Devise a way to find an element in the rotated array in O(log n) time. Input : arr[] = {5, 6, 7, 8, 9, 10, 1, 2, 3};
key = 3
Output : Found at index 8
The idea is to find the pivot point, divide the array in two sub-arrays and call binary search.
The main idea for finding pivot is â for a sorted (in increasing order) and pivoted array, pivot element is the only element for which next element to it is smaller than it.
Using above criteria and binary search methodology we can get pivot element in O(logn) time
Program to search an element in a sorted and pivoted array
int findPivot(int[], int, int);
int binarySearch(int[], int, int, int);
/* Searches an element key in a pivoted sorted array arrp[]
of size n */
int pivotedBinarySearch(int arr[], int n, int key) {
int pivot = findPivot(arr, 0, n - 1);
// If we didn't find a pivot, then array is not rotated at all
if (pivot == -1)
return binarySearch(arr, 0, n - 1, key);
// If we found a pivot, then first compare with pivot and then
// search in two subarrays around pivot
if (arr[pivot] == key)
return pivot;
if (arr[0] <= key)
return binarySearch(arr, 0, pivot - 1, key);
return binarySearch(arr, pivot + 1, n - 1, key);
}
/* Function to get pivot. For array 3, 4, 5, 6, 1, 2 it returns
3 (index of 6) */
int findPivot(int arr[], int low, int high) {
// base cases
if (high < low) return -1;
if (high == low) return low;
int mid = (low + high) / 2; /*low + (high - low)/2;*/
if (mid < high && arr[mid] > arr[mid + 1])
return mid;
if (mid > low && arr[mid] < arr[mid - 1])
return (mid - 1);
if (arr[low] >= arr[mid])
return findPivot(arr, low, mid - 1);
return findPivot(arr, mid + 1, high);
}
/* Standard Binary Search function*/
int binarySearch(int arr[], int low, int high, int key) {
if (high < low)
return -1;
int mid = (low + high) / 2; /*low + (high - low)/2;*/
if (key == arr[mid])
return mid;
if (key > arr[mid])
return binarySearch(arr, (mid + 1), high, key);
return binarySearch(arr, low, (mid - 1), key);
}
/* main program to check above functions */
int main() {
// Let us search 3 in below array
int arr1[] = {
5,
6,
7,
8,
9,
10,
1,
2,
3
};
int n = sizeof(arr1) / sizeof(arr1[0]);
int key = 3;
printf("Index of the element is : %d",
pivotedBinarySearch(arr1, n, key));
return 0;
}
Output:
Index of the element is : 8
What are the differences between throw and throws in Java?
throw In Java: throw is a keyword in java which is used to throw an exception manually. Using throw keyword, you can throw an exception from any method or block. But, that exception must be of type java.lang.Throwable class or itâs sub classes. Below example shows how to throw an exception using throw keyword.
class ThrowAndThrowsExample {
void method() throws Exception {
Exception e = new Exception();
throw e; //throwing an exception using 'throw'
}
}
throws In Java:
throws is also a keyword in java which is used in the method signature to indicate that this method may throw mentioned exceptions. The caller to such methods must handle the mentioned exceptions either using try-catch blocks or using throws keyword.
Below is the syntax for using throws keyword.
return_type method_name(parameter_list) throws exception_list {
//some statements
}
Below is the example which shows how to use throws keyword.
class ThrowsExample {
void methodOne() throws SQLException {
//This method may throw SQLException
}
void methodTwo() throws IOException {
//This method may throw IOException
}
void methodThree() throws ClassNotFoundException {
//This method may throw ClassNotFoundException
}
}
What are various access modifiers in JAVA?
There are four access modifiers in java that control visibility:
- Private: It is visible to members to same class only
- Public: It is visible everywhere
- Protected: Visible only to the package and subclasses
- Default: If there is no access specifier mentioned explicitly it is considered as default and it is visible only to same package.
Write a C function to count number of nodes in a given singly linked list.
Solution:
1) Initialize count as 0
2) Initialize a node pointer, current = head.
3) Do following while current is not NULL
a) current = current -> next
b) count++;
4) Return count
C Code to get count of number of nodes in a linked list:
int getCount(struct Node * head) { int count = 0; // Initialize count struct Node * current = head; // Initialize current while (current != NULL) { count++; current = current - > next; } return count; }